Plots#
- Related modules
- Detailed examples
- Additional imports
In addition to the user-guide imports, the pages (Plots, Plotting Primer and Customising MQR plots) also require the following imports.
from matplotlib import pyplot as plt
import seaborn as sns
Basics#
The summaries and example notebooks use two libraries: matplotlib
and seaborn
.
matplotlib provides all the basic plotting tools, like figures and axes. It also provides various plot types (see Elements section).
seaborn builds on matplotlib to provide more sophisticated plots, mostly related to visualising statistics. It also provides alternatives to some matplotlib plots.
Getting help#
Both libraries provide excellent documentation, detailing how to use the libraries and showing examples. There are two quick ways to access documentation.
In the Jupyter notebook, type the name of the function and parentheses, place the cursor somewhere between the parentheses, then type
shift-tab
. Eg. typesns.boxplot(...)
, then while the cursor is somewhere in(...)
typeshift-tab
.Go to the websites below, and use the search function on their website to find a function, example, etc.
matplotlib https://matplotlib.org/stable/
seaborn https://seaborn.pydata.org/index.html
Matplotlib figures and axes#
Matplotlib plots are based on figures and axes. A figure holds one or more axes. For more info on figures and axes, see https://matplotlib.org/stable/users/explain/axes/axes_intro.html.
Create a figure with a given size like this:
fig, ax = plt.subplots(figsize=(6, 3))
plt.show(fig)
plt.close(fig)
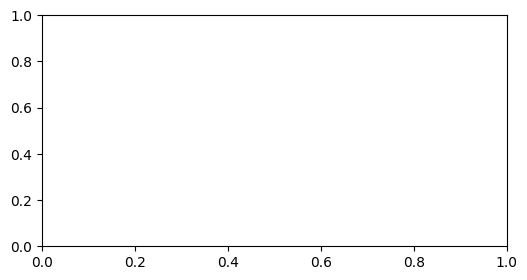
In this guide and the example notebooks, the axes and figure variables are stored and used explicitly.
For example, ax.plot(...)
is usually used to draw a line, and
sns.histplot(..., ax=ax)
would be used to create a histogram.
Lots of examples on the internet will not assign the result of calling subplots
.
If you do not specify an axis to plot onto, matplotlib will plot onto the most recently created axis.
We suggest keeping track of the axes you create by assigning them to variables like we do in this guide,
then plotting into them explicitly.
Explicit code is easier to read and easier to fix when there are problems.
Figure context manager#
MQR provides a wrapper around subplot creation.
The wrapper is written as a with
-block called Figure
.
The with
construct is a python feature called a context manager.
It helps automatically initialise and then destroy resources (the figure in this case).
The Figure
block creates a figure with the arguments supplied,
and does a few other things to automate common actions to make nice looking figures in notebooks.
The code it executes is here: .
You can use either construct: fig, ax = matplotlib.subplots(...)
or with Figure(...) as (fig, ax): ...
.
with Figure() as (fig, ax):
pass
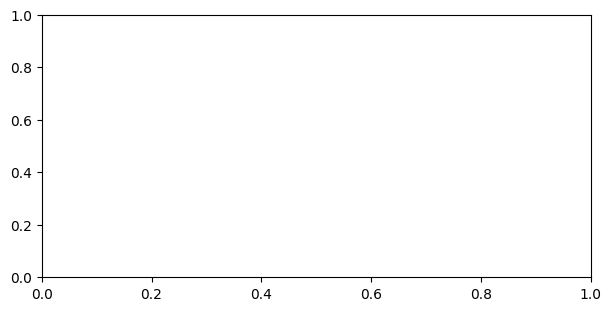
MQR notebook tools#
The module mqr.nbtools
can show matplotlib plots alongside other objects.
To capture the matplotlib output, use mqr.nbtools.grab_figure
, as below.
The result of grab_figure
is an HTML image element with the figure’s data embedded directly as a png image.
After grabbing the image data, the figure is destroyed and matplotlib will not render it (unless suppress=False
).
Instead, call display on the returned HTML, or combine it with other HTML (like in mqr.nbtools.hstack
, etc).
For multiple plots, use matplotlib.pyplot.subplots
,
because it has comprehensive features for showing plots next to each other (like shared axes and height/width ratios).
Grabbing a figure is useful for placing figures next to DataFrame
s and other objects that implement _repr_html_
.
line_xs = np.linspace(0, 10)
line_ys = line_xs ** 2
point_xs = np.linspace(0, 10, 11)
point_ys = point_xs ** 2
with Figure(4, 4) as (fig, ax):
ax.plot(
line_xs, # x values
line_ys, # y values
linewidth=0.5, color='C0')
ax.plot(
point_xs,
point_ys,
linewidth=0, color='C1', marker='o', fillstyle='none')
ax.set_xlabel('x')
ax.set_ylabel('y')
plot = mqr.nbtools.grab_figure(fig)
table_data = pd.DataFrame(
data={'x': np.linspace(0, 10, 11),
'y': np.linspace(0, 10, 11)**2})
mqr.nbtools.vstack(
'#### Parabola',
mqr.nbtools.hstack(
plot,
table_data
)
)
Parabola
x | y | |
---|---|---|
0 | 0.0 | 0.0 |
1 | 1.0 | 1.0 |
2 | 2.0 | 4.0 |
3 | 3.0 | 9.0 |
4 | 4.0 | 16.0 |
5 | 5.0 | 25.0 |
6 | 6.0 | 36.0 |
7 | 7.0 | 49.0 |
8 | 8.0 | 64.0 |
9 | 9.0 | 81.0 |
10 | 10.0 | 100.0 |
Directory#
MQR constructs the following common plots for convenience.
All plots that require multiple axes flatten the ax
argument before use,
so any dimensions that multiply to give the value of “No. axes” below will work,
excluding mqr.plot.correlation.matrix
, which must be exactly N by N.
Some common plots are not listed here, because there are already implementations in matplotlib and seaborn. For example, MQR does not implement scatter plots, line plots, box plots or histograms. The Plotting primer shows how to construct those directly from matplotlib or seaborn. matplotlib plot types and seaborn examples for examples of other plots.
Process
Function |
No. axes |
Description |
---|---|---|
1 |
Fishbone/Ishikawa diagram |
|
N by N |
Matrix of scatter plots, histrograms and correlation statistics |
|
1 |
Interval and points showing a confidence interval and hypothesised value |
|
3 |
For a sample: histogram, boxplot and confidence interval of the mean |
|
1 |
Probability density of a process estimating its yield |
|
1 |
Shaded region representing a tolerance |
|
1 |
For a process: a histrogram, pdf and tolerance overalyed, showing capability. |
Regression
Function |
No. axes |
Description |
---|---|---|
4 |
For an ols result: a tableau of the above three plots, and a probability plot |
|
1 |
For an OLS result: bar graphs of an influence statistic |
|
1 |
For OLS residuals: normal probability plot |
|
1 |
For OLS residuals: histogram with overlayed density |
|
1 |
For OLS residuals: residuals vs. observations |
|
1 |
For OLS residuals: residuals vs. fitted values |
|
1 |
For OLS residuals: residuals vs. factor data |
ANOVA
Function |
No. axes |
Description |
---|---|---|
N |
Main effects for experimental data, for N effects |
|
N |
Interactions between independent variables, for N interactions |
|
1 |
Means for each treatment combinations, as confidence bars |
Measurement system analysis
Function |
No. axes |
Description |
---|---|---|
6 |
A tableau of the above six plots |
|
1 |
For a GRR: bar graph of percent contributions from variances |
|
1 |
For a GRR: box-plot of measurements by part |
|
1 |
For a GRR: box-plot of measurments by operator |
|
1 |
For a GRR: Xbar-chart for each operator |
|
1 |
For a GRR: R-chart for each operator |
|
1 |
For a GRR: the part-operator interactions |
Statistical process control
Function |
No. axes |
Description |
---|---|---|
1 |
Chart of a monitored statistics |
|
1 |
Chart overlay for out-of-control statistics |
|
1 |
For a sample: an operating characteristic curve |
Other tools
Class |
Description |
---|---|
A context manager that wraps a call to |
|
A routine that renders a figure as png into an HTML component, then closes the figure |